Features
Introduction
Introduction
The TransposedGrid control display data using a transposed layout, where columns represent data items and rows represent item properties.
Features
TransposedGrid:
Item0
Item1
Item2
Item3
Item4
Item5
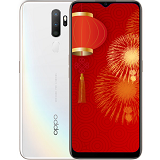
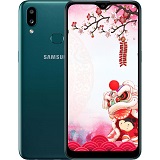
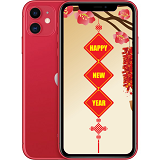
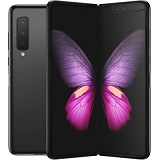
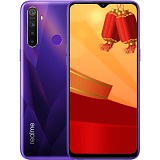
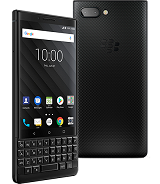
OPPO A5 (2020) 64GB
Samsung Galaxy A10s
iPhone 11 64GB
Samsung Galaxy Fold
Realme 5 (3GB/64GB)
BlackBerry KEY2
★★★★
★★
★★★★★
★★★★★
★★★☆
★★★★☆
$199.00
$155.00
$999.00
$1,898.00
$166.00
$711.00
TFT, 6.5", HD+
IPS TFT, 6.2", HD+
IPS LCD, 6.1", Liquid Retina
Dynamic AMOLED 7.3" & Super AMOLED 4.6", QuadHD(2K)
IPS LCD, 6.5", HD+
IPS LCD, 4.5", Full HD
Main: 12 MP & Others: 8 MP, 2 MP, 2 MP
Main: 13 MP & Second: 2 MP
Main: 12 MP & Second: 12 MP
Main: 12 MP & Others: 12 MP, 16 MP
Main: 12 MP & Others: 8 MP, 2 MP, 2 MP
Main: 12 MP & Second: 12 MP
Android 9.0 (Pie)
Android 9.0 (Pie)
iOS 13
Android 9.0 (Pie)
Android 9.0 (Pie)
Android 8.1 (Oreo)
Snapdragon 665 8 cores
MediaTek MT6762 8 cores (Helio P22)
Apple A13 Bionic 6 cores
Snapdragon 855 8 cores
Snapdragon 665 8 cores
Snapdragon 660 8 cores
3GB
2GB
4GB
12GB
3GB
6GB
64GB
32GB
64GB
512GB
64GB
64GB
2 Nano SIM, Support 4G
2 Nano SIM, Support 4G
1 eSIM & 1 Nano SIM, Support 4G
1 eSIM & 1 Nano SIM, Support 4G
2 Nano SIM, Support 4G
2 Nano SIM, Support 4G
5,000mAh
4,000mAh
3,110mAh
4,380mAh
5,000mAh
3,500mAh
Model
Rating
Price
Screen
Camera
OS
CPU
RAM
ROM
SIM
Battery
0
FlexGrid:
Model
Rating
Price
Screen
Camera
OS
CPU
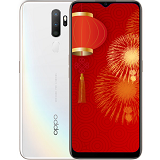
OPPO A5 (2020) 64GB
★★★★
$199.00
TFT, 6.5", HD+
Main: 12 MP & Others: 8 MP, 2 MP, 2 MP
Android 9.0 (Pie)
Snapdragon 665 8 cores
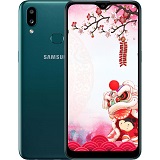
Samsung Galaxy A10s
★★
$155.00
IPS TFT, 6.2", HD+
Main: 13 MP & Second: 2 MP
Android 9.0 (Pie)
MediaTek MT6762 8 cores (Helio P22)
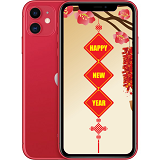
iPhone 11 64GB
★★★★★
$999.00
IPS LCD, 6.1", Liquid Retina
Main: 12 MP & Second: 12 MP
iOS 13
Apple A13 Bionic 6 cores
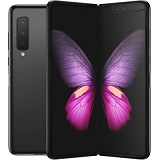
Samsung Galaxy Fold
★★★★★
$1,898.00
Dynamic AMOLED 7.3" & Super AMOLED 4.6", QuadHD(2K)
Main: 12 MP & Others: 12 MP, 16 MP
Android 9.0 (Pie)
Snapdragon 855 8 cores
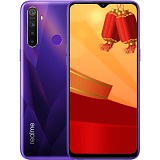
Realme 5 (3GB/64GB)
★★★☆
$166.00
IPS LCD, 6.5", HD+
Main: 12 MP & Others: 8 MP, 2 MP, 2 MP
Android 9.0 (Pie)
Snapdragon 665 8 cores
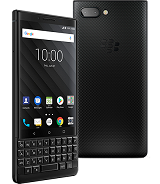
BlackBerry KEY2
★★★★☆
$711.00
IPS LCD, 4.5", Full HD
Main: 12 MP & Second: 12 MP
Android 8.1 (Oreo)
Snapdragon 660 8 cores
Model
Rating
Price
Screen
Camera
OS
CPU
0
Description
This sample uses TransposedGrid control to display products on the grid columns, and their properties on the grid rows.
Transposed layouts are especially useful for comparing items, or displaying few data items which each items has many properties.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | using System; using System.Collections.Generic; using System.Linq; using Microsoft.AspNetCore.Mvc; using TransposedGridExplorer.Models; using C1.Web.Mvc; using C1.Web.Mvc.Serialization; #if !NETCORE30 using Microsoft.AspNetCore.Http.Internal; #endif using Microsoft.Extensions.Primitives; using Microsoft.AspNetCore.Http; namespace TransposedGridExplorer.Controllers { public partial class TransposedGridController : Controller { public ActionResult Index() { return View(ProductSheet.GetData()); } } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 | @using C1.Web.Mvc.Grid @model IEnumerable< ProductSheet > < h4 class = "semi-bold" > TransposedGrid: </ h4 > < c1-transposed-grid id = "theTransposedGrid" auto-generate-rows = "false" class = "product-sheet-grid" is-read-only = "true" headers-visibility = "Row" item-formatter = "formatItem" loaded-rows = "loadedRows" height = "615" > < c1-items-source source-collection = "Model" ></ c1-items-source > < c1-transposed-grid-row binding = "Image" header = " " align = "center" width = "80" ></ c1-transposed-grid-row > < c1-transposed-grid-row binding = "Name" header = "Model" align = "center" word-wrap = "true" ></ c1-transposed-grid-row > < c1-transposed-grid-row binding = "Rating" header = "Rating" align = "center" format = "n1" width = "130" ></ c1-transposed-grid-row > < c1-transposed-grid-row binding = "Price" header = "Price" align = "center" format = "c2" width = "120" ></ c1-transposed-grid-row > < c1-transposed-grid-row binding = "Screen" header = "Screen" align = "center" word-wrap = "true" ></ c1-transposed-grid-row > < c1-transposed-grid-row binding = "Camera" header = "Camera" align = "center" word-wrap = "true" ></ c1-transposed-grid-row > < c1-transposed-grid-row binding = "OS" header = "OS" align = "center" word-wrap = "true" ></ c1-transposed-grid-row > < c1-transposed-grid-row binding = "CPU" header = "CPU" align = "center" word-wrap = "true" width = "150" ></ c1-transposed-grid-row > < c1-transposed-grid-row binding = "RAM" header = "RAM" align = "center" format = "n0" width = "80" ></ c1-transposed-grid-row > < c1-transposed-grid-row binding = "ROM" header = "ROM" align = "center" format = "n0" width = "80" ></ c1-transposed-grid-row > < c1-transposed-grid-row binding = "SIM" header = "SIM" align = "center" word-wrap = "true" ></ c1-transposed-grid-row > < c1-transposed-grid-row binding = "Battery" header = "Battery" align = "center" format = "n0" width = "80" ></ c1-transposed-grid-row > </ c1-transposed-grid > < h4 class = "semi-bold" > FlexGrid: </ h4 > < c1-flex-grid id = "theFlexGrid" auto-generate-columns = "false" class = "product-sheet-grid" is-read-only = "true" item-formatter = "formatItem" height = "350" default-row-size = "50" default-column-size = "210" > < c1-items-source source-collection = "Model" ></ c1-items-source > < c1-flex-grid-column binding = "Image" header = " " align = "center" width = "80" ></ c1-flex-grid-column > < c1-flex-grid-column binding = "Name" header = "Model" align = "center" word-wrap = "true" ></ c1-flex-grid-column > < c1-flex-grid-column binding = "Rating" header = "Rating" align = "center" format = "n1" width = "130" ></ c1-flex-grid-column > < c1-flex-grid-column binding = "Price" header = "Price" align = "center" format = "c2" width = "120" ></ c1-flex-grid-column > < c1-flex-grid-column binding = "Screen" header = "Screen" align = "center" word-wrap = "true" ></ c1-flex-grid-column > < c1-flex-grid-column binding = "Camera" header = "Camera" align = "center" word-wrap = "true" ></ c1-flex-grid-column > < c1-flex-grid-column binding = "OS" header = "OS" align = "center" word-wrap = "true" ></ c1-flex-grid-column > < c1-flex-grid-column binding = "CPU" header = "CPU" align = "center" word-wrap = "true" width = "150" ></ c1-flex-grid-column > < c1-flex-grid-column binding = "RAM" header = "RAM" align = "center" format = "n0" width = "80" ></ c1-flex-grid-column > < c1-flex-grid-column binding = "ROM" header = "ROM" align = "center" format = "n0" width = "80" ></ c1-flex-grid-column > < c1-flex-grid-column binding = "SIM" header = "SIM" align = "center" word-wrap = "true" ></ c1-flex-grid-column > < c1-flex-grid-column binding = "Battery" header = "Battery" align = "center" format = "n0" width = "80" ></ c1-flex-grid-column > </ c1-flex-grid > < style type = "text/css" > .product-sheet-grid { max-height: 2000px; } .product-sheet-grid .wj-rowheaders .wj-cell { text-transform: uppercase; font-size: 80%; } .product-sheet-grid .wj-cell { padding: 4px; border-bottom: none; } .product-sheet-grid .wj-cell span.rating { font-size: x-large; color: #e7711b; margin-right: 0; } .product-sheet-grid .wj-cell span.price { font-weight: bold; color: #d0021b; } .product-sheet-grid img { max-width: 100%; max-height: 100%; } .star { font-size: x-large; width: auto; display: inline-block; color: gray; } .star.half:after { content: '\2605' ; color: #e7711b; margin-left: -20px; width: 10px; position: absolute; overflow: hidden; } </ style > @section Scripts{ <script> function loadedRows(grid) { let g = grid; g.columns.defaultSize = 210; setTimeout( function () { g.autoSizeColumn(0, true , 30); g.autoSizeRows(); g.rows[0].height = 170; }); } function formatItem(panel, r, c, cell) { if (panel.cellType == 1) { let binding = panel.rows[r].binding || panel.columns[c].binding; switch (binding) { case 'Image' : cell.innerHTML = '<img src="' + '@Url.Content("~/")' + cell.textContent + '" draggable="false"/>' ; break ; case 'Name' : cell.innerHTML = '<b>' + cell.innerHTML + '</b>' ; break ; case 'Price' : cell.innerHTML = '<span class="price">' + cell.innerHTML + '</span>' ; break ; // rating as stars case 'Rating' : let rating = panel.getCellData(r, c, false ) * 1, stars = Math.floor(rating), html = new Array(stars + 1).join( '★' ); html = '<span class="rating">' + html + '</span>' ; if (rating > stars) { html += '<span class="star half">☆</span>' ; } cell.innerHTML = html; break ; case 'RAM' : case 'ROM' : cell.textContent += "GB" ; break ; case 'Battery' : cell.textContent += "mAh" ; break ; } } } </script> } @section Summary{ < p > @Html .Raw(TransposedGridRes.Index_Text0)</ p > } @section Description{ < p > @Html .Raw(TransposedGridRes.Index_Text1)</ p > } |